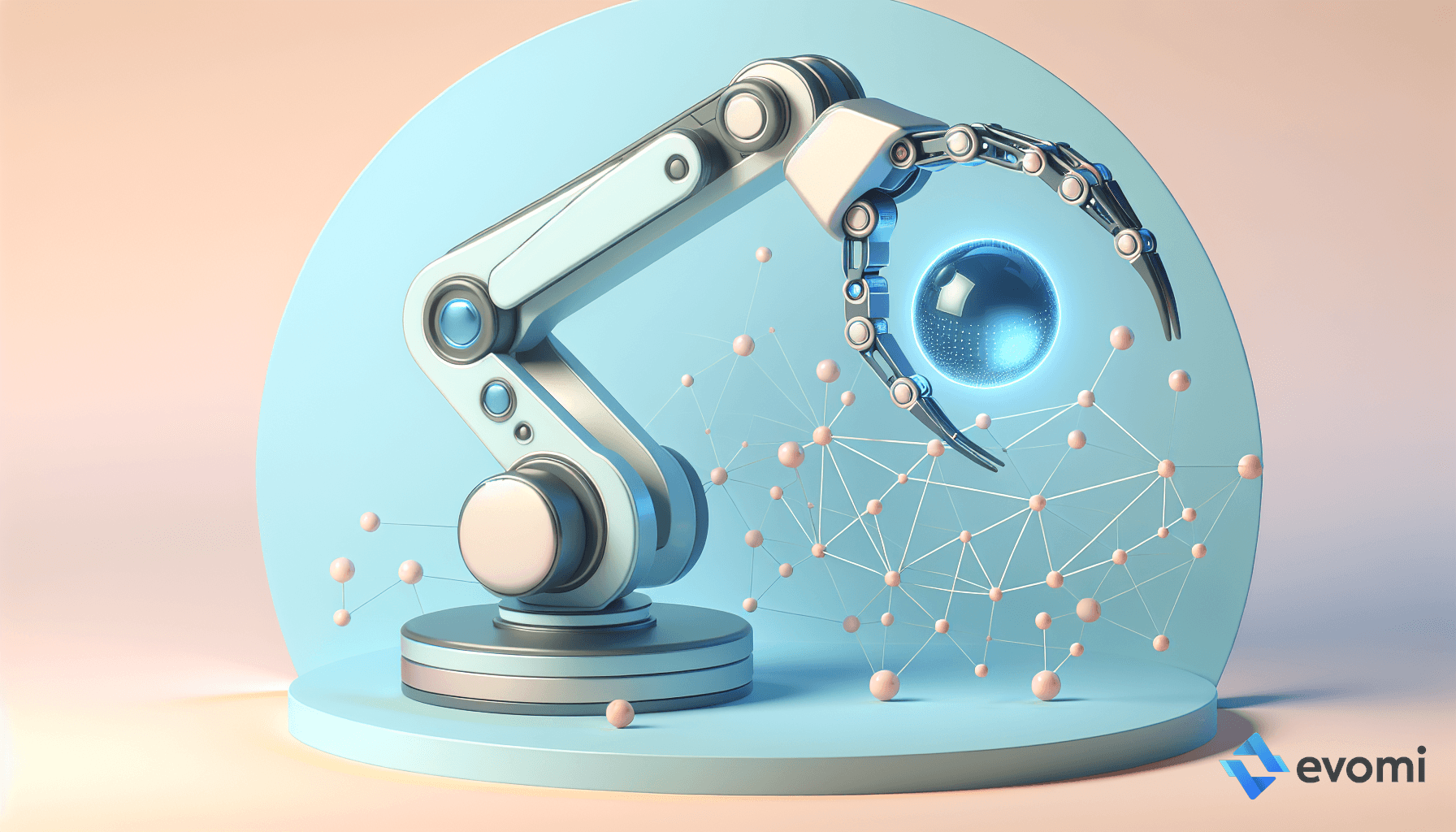
Master Automated Web Scraping in Any Programming Language: A Comprehensive Guide
Web scraping has become an essential tool for businesses and researchers alike, enabling them to extract valuable data from websites quickly and efficiently. Whether you're looking to gather market insights, monitor competitor pricing, or collect data for machine learning models, automated web scraping can be a game-changer. In this comprehensive guide, we'll explore how to master web scraping techniques using various programming languages, ensuring you can choose the approach that best fits your skillset and project requirements.
Understanding the Basics of Web Scraping
Before diving into specific programming languages, it's crucial to grasp the fundamental concepts of web scraping. At its core, web scraping involves sending HTTP requests to web servers, receiving HTML responses, and parsing the content to extract desired information. This process can be broken down into several key steps:
Sending HTTP requests to target websites
Handling the server's response
Parsing the HTML content
Extracting relevant data
Storing or processing the extracted information
Understanding these steps will help you approach web scraping projects more effectively, regardless of the programming language you choose. It's also important to be aware of ethical considerations and legal implications when scraping websites. Always respect robots.txt files, implement rate limiting to avoid overwhelming servers, and ensure you're not violating any terms of service.
Python: The Go-To Language for Web Scraping
Python has long been the preferred language for web scraping due to its simplicity and powerful libraries. Libraries like Beautiful Soup and Scrapy have made it easier than ever to extract data from web pages. Here's a basic example of how you might use Python with the requests and Beautiful Soup libraries to scrape a website:
import requestsfrom bs4 import BeautifulSoupurl = 'https://example.com'response = requests.get(url)soup = BeautifulSoup(response.text, 'html.parser')# Extract all paragraph textsparagraphs = soup.find_all('p')for p in paragraphs: print(p.text)
Python's popularity in the data science and machine learning communities also makes it an excellent choice for projects that involve analyzing the scraped data. With libraries like pandas and numpy, you can easily process and visualize the information you've collected.
JavaScript: Scraping in the Browser and Beyond
JavaScript has become increasingly popular for web scraping, especially with the rise of Node.js for server-side scripting. One of the main advantages of using JavaScript is its ability to interact with dynamic web pages that load content asynchronously. Libraries like Puppeteer and Cheerio have made it possible to automate browser interactions and parse HTML with ease. Here's a simple example using Node.js and Cheerio:
const axios = require('axios');const cheerio = require('cheerio');async function scrapeWebsite() { const url = 'https://example.com'; const response = await axios.get(url); const $ = cheerio.load(response.data); // Extract all heading texts $('h1, h2, h3').each((index, element) => { console.log($(element).text()); });}scrapeWebsite();
JavaScript's asynchronous nature makes it well-suited for handling multiple requests concurrently, which can significantly speed up large-scale scraping operations. Additionally, its widespread use in web development means that many developers are already familiar with the language, making it a natural choice for web scraping projects.
Ruby: Elegant Scraping with Nokogiri
Ruby, known for its elegant syntax and developer-friendly ecosystem, offers powerful web scraping capabilities through libraries like Nokogiri. Ruby's simplicity and readability make it an excellent choice for those who prioritize clean, maintainable code. Here's a brief example of web scraping using Ruby and Nokogiri:
require 'nokogiri'require 'open-uri'url = 'https://example.com'doc = Nokogiri::HTML(URI.open(url))# Extract all link texts and URLsdoc.css('a').each do |link| puts "#{link.text} - #{link['href']}"end
Ruby's extensive gem ecosystem provides additional tools for handling various aspects of web scraping, such as handling cookies, managing sessions, and dealing with JavaScript-rendered content. The language's focus on developer happiness also makes it a joy to work with for long-term scraping projects.
Overcoming Common Web Scraping Challenges
Regardless of the programming language you choose, you're likely to encounter some common challenges when scraping websites. Here are some tips to help you navigate these obstacles:
Handling dynamic content: Many modern websites use JavaScript to load content dynamically. To scrape these sites effectively, you may need to use headless browsers or tools that can execute JavaScript, such as Selenium or Puppeteer.
Dealing with rate limiting and IP blocks: Websites often implement measures to prevent excessive scraping. To avoid being blocked, consider using proxy servers, implementing delays between requests, and rotating user agents. At Evomi, we offer reliable proxy solutions that can help you overcome these challenges while ensuring compliance with ethical scraping practices.
Parsing complex HTML structures: Some websites have intricate HTML structures that can be difficult to navigate. Familiarize yourself with advanced CSS selectors and XPath queries to extract data from these complex layouts more effectively.
Handling authentication and sessions: For websites that require login, you'll need to manage cookies and maintain session state. Most programming languages have libraries or modules to help with this, such as the requests-html library for Python.
Scaling your scraping operations: As your data collection needs grow, you may need to scale your scraping operations. Consider using distributed systems, message queues, or cloud-based solutions to handle large-scale scraping tasks efficiently.
Ethical Considerations and Best Practices
While web scraping can be an incredibly powerful tool for data collection, it's crucial to approach it ethically and responsibly. Here are some best practices to keep in mind:
Respect robots.txt: Always check and adhere to the rules specified in a website's robots.txt file. This file outlines which parts of the site can be crawled and at what frequency.
Implement rate limiting: Avoid overwhelming servers by limiting the rate of your requests. This not only helps maintain good relationships with website owners but also reduces the likelihood of your IP being blocked.
Identify your scraper: Use a custom user agent that identifies your scraper and provides contact information. This allows website owners to reach out if they have concerns about your scraping activities.
Be mindful of copyright: Ensure that you're not violating copyright laws when scraping and using content from websites. Some data may be protected, and it's essential to understand the legal implications of your scraping activities.
Consider API alternatives: Before scraping a website, check if they offer an API for data access. Using an official API is often more reliable and respectful than scraping.
At Evomi, we understand the importance of ethical web scraping and provide solutions that help businesses collect data responsibly. Our proxy services are designed to support legitimate data collection efforts while maintaining compliance with best practices and legal requirements.
Conclusion: Choosing the Right Approach for Your Project
Mastering automated web scraping is a valuable skill that can significantly enhance your data collection capabilities. Whether you choose Python, JavaScript, Ruby, or any other programming language, the key is to understand the fundamental concepts and challenges involved in web scraping.
When selecting a language and approach for your web scraping project, consider factors such as your team's expertise, the complexity of the websites you'll be scraping, and the scale of your data collection needs. Remember that the most effective web scraping solutions often combine multiple tools and techniques to overcome various challenges.
As you embark on your web scraping journey, don't hesitate to explore the various libraries and frameworks available in your chosen language. Experiment with different approaches, and always prioritize ethical scraping practices. With the right tools and mindset, you'll be well-equipped to tackle even the most complex web scraping projects and unlock valuable insights from the vast sea of online data.
If you're looking for reliable proxy solutions to support your web scraping efforts, consider giving Evomi a try. Our range of residential, mobile, and datacenter proxies can help you overcome common scraping obstacles while ensuring high-quality, ethical data collection. With competitive pricing starting at just $0.35 per GB for datacenter proxies and $2.15 per GB for residential and mobile proxies, we offer cost-effective solutions for businesses of all sizes. Plus, our free trial allows you to experience the benefits of our services risk-free. Take your web scraping capabilities to the next level with Evomi's robust proxy infrastructure.
Master Automated Web Scraping in Any Programming Language: A Comprehensive Guide
Web scraping has become an essential tool for businesses and researchers alike, enabling them to extract valuable data from websites quickly and efficiently. Whether you're looking to gather market insights, monitor competitor pricing, or collect data for machine learning models, automated web scraping can be a game-changer. In this comprehensive guide, we'll explore how to master web scraping techniques using various programming languages, ensuring you can choose the approach that best fits your skillset and project requirements.
Understanding the Basics of Web Scraping
Before diving into specific programming languages, it's crucial to grasp the fundamental concepts of web scraping. At its core, web scraping involves sending HTTP requests to web servers, receiving HTML responses, and parsing the content to extract desired information. This process can be broken down into several key steps:
Sending HTTP requests to target websites
Handling the server's response
Parsing the HTML content
Extracting relevant data
Storing or processing the extracted information
Understanding these steps will help you approach web scraping projects more effectively, regardless of the programming language you choose. It's also important to be aware of ethical considerations and legal implications when scraping websites. Always respect robots.txt files, implement rate limiting to avoid overwhelming servers, and ensure you're not violating any terms of service.
Python: The Go-To Language for Web Scraping
Python has long been the preferred language for web scraping due to its simplicity and powerful libraries. Libraries like Beautiful Soup and Scrapy have made it easier than ever to extract data from web pages. Here's a basic example of how you might use Python with the requests and Beautiful Soup libraries to scrape a website:
import requestsfrom bs4 import BeautifulSoupurl = 'https://example.com'response = requests.get(url)soup = BeautifulSoup(response.text, 'html.parser')# Extract all paragraph textsparagraphs = soup.find_all('p')for p in paragraphs: print(p.text)
Python's popularity in the data science and machine learning communities also makes it an excellent choice for projects that involve analyzing the scraped data. With libraries like pandas and numpy, you can easily process and visualize the information you've collected.
JavaScript: Scraping in the Browser and Beyond
JavaScript has become increasingly popular for web scraping, especially with the rise of Node.js for server-side scripting. One of the main advantages of using JavaScript is its ability to interact with dynamic web pages that load content asynchronously. Libraries like Puppeteer and Cheerio have made it possible to automate browser interactions and parse HTML with ease. Here's a simple example using Node.js and Cheerio:
const axios = require('axios');const cheerio = require('cheerio');async function scrapeWebsite() { const url = 'https://example.com'; const response = await axios.get(url); const $ = cheerio.load(response.data); // Extract all heading texts $('h1, h2, h3').each((index, element) => { console.log($(element).text()); });}scrapeWebsite();
JavaScript's asynchronous nature makes it well-suited for handling multiple requests concurrently, which can significantly speed up large-scale scraping operations. Additionally, its widespread use in web development means that many developers are already familiar with the language, making it a natural choice for web scraping projects.
Ruby: Elegant Scraping with Nokogiri
Ruby, known for its elegant syntax and developer-friendly ecosystem, offers powerful web scraping capabilities through libraries like Nokogiri. Ruby's simplicity and readability make it an excellent choice for those who prioritize clean, maintainable code. Here's a brief example of web scraping using Ruby and Nokogiri:
require 'nokogiri'require 'open-uri'url = 'https://example.com'doc = Nokogiri::HTML(URI.open(url))# Extract all link texts and URLsdoc.css('a').each do |link| puts "#{link.text} - #{link['href']}"end
Ruby's extensive gem ecosystem provides additional tools for handling various aspects of web scraping, such as handling cookies, managing sessions, and dealing with JavaScript-rendered content. The language's focus on developer happiness also makes it a joy to work with for long-term scraping projects.
Overcoming Common Web Scraping Challenges
Regardless of the programming language you choose, you're likely to encounter some common challenges when scraping websites. Here are some tips to help you navigate these obstacles:
Handling dynamic content: Many modern websites use JavaScript to load content dynamically. To scrape these sites effectively, you may need to use headless browsers or tools that can execute JavaScript, such as Selenium or Puppeteer.
Dealing with rate limiting and IP blocks: Websites often implement measures to prevent excessive scraping. To avoid being blocked, consider using proxy servers, implementing delays between requests, and rotating user agents. At Evomi, we offer reliable proxy solutions that can help you overcome these challenges while ensuring compliance with ethical scraping practices.
Parsing complex HTML structures: Some websites have intricate HTML structures that can be difficult to navigate. Familiarize yourself with advanced CSS selectors and XPath queries to extract data from these complex layouts more effectively.
Handling authentication and sessions: For websites that require login, you'll need to manage cookies and maintain session state. Most programming languages have libraries or modules to help with this, such as the requests-html library for Python.
Scaling your scraping operations: As your data collection needs grow, you may need to scale your scraping operations. Consider using distributed systems, message queues, or cloud-based solutions to handle large-scale scraping tasks efficiently.
Ethical Considerations and Best Practices
While web scraping can be an incredibly powerful tool for data collection, it's crucial to approach it ethically and responsibly. Here are some best practices to keep in mind:
Respect robots.txt: Always check and adhere to the rules specified in a website's robots.txt file. This file outlines which parts of the site can be crawled and at what frequency.
Implement rate limiting: Avoid overwhelming servers by limiting the rate of your requests. This not only helps maintain good relationships with website owners but also reduces the likelihood of your IP being blocked.
Identify your scraper: Use a custom user agent that identifies your scraper and provides contact information. This allows website owners to reach out if they have concerns about your scraping activities.
Be mindful of copyright: Ensure that you're not violating copyright laws when scraping and using content from websites. Some data may be protected, and it's essential to understand the legal implications of your scraping activities.
Consider API alternatives: Before scraping a website, check if they offer an API for data access. Using an official API is often more reliable and respectful than scraping.
At Evomi, we understand the importance of ethical web scraping and provide solutions that help businesses collect data responsibly. Our proxy services are designed to support legitimate data collection efforts while maintaining compliance with best practices and legal requirements.
Conclusion: Choosing the Right Approach for Your Project
Mastering automated web scraping is a valuable skill that can significantly enhance your data collection capabilities. Whether you choose Python, JavaScript, Ruby, or any other programming language, the key is to understand the fundamental concepts and challenges involved in web scraping.
When selecting a language and approach for your web scraping project, consider factors such as your team's expertise, the complexity of the websites you'll be scraping, and the scale of your data collection needs. Remember that the most effective web scraping solutions often combine multiple tools and techniques to overcome various challenges.
As you embark on your web scraping journey, don't hesitate to explore the various libraries and frameworks available in your chosen language. Experiment with different approaches, and always prioritize ethical scraping practices. With the right tools and mindset, you'll be well-equipped to tackle even the most complex web scraping projects and unlock valuable insights from the vast sea of online data.
If you're looking for reliable proxy solutions to support your web scraping efforts, consider giving Evomi a try. Our range of residential, mobile, and datacenter proxies can help you overcome common scraping obstacles while ensuring high-quality, ethical data collection. With competitive pricing starting at just $0.35 per GB for datacenter proxies and $2.15 per GB for residential and mobile proxies, we offer cost-effective solutions for businesses of all sizes. Plus, our free trial allows you to experience the benefits of our services risk-free. Take your web scraping capabilities to the next level with Evomi's robust proxy infrastructure.
Master Automated Web Scraping in Any Programming Language: A Comprehensive Guide
Web scraping has become an essential tool for businesses and researchers alike, enabling them to extract valuable data from websites quickly and efficiently. Whether you're looking to gather market insights, monitor competitor pricing, or collect data for machine learning models, automated web scraping can be a game-changer. In this comprehensive guide, we'll explore how to master web scraping techniques using various programming languages, ensuring you can choose the approach that best fits your skillset and project requirements.
Understanding the Basics of Web Scraping
Before diving into specific programming languages, it's crucial to grasp the fundamental concepts of web scraping. At its core, web scraping involves sending HTTP requests to web servers, receiving HTML responses, and parsing the content to extract desired information. This process can be broken down into several key steps:
Sending HTTP requests to target websites
Handling the server's response
Parsing the HTML content
Extracting relevant data
Storing or processing the extracted information
Understanding these steps will help you approach web scraping projects more effectively, regardless of the programming language you choose. It's also important to be aware of ethical considerations and legal implications when scraping websites. Always respect robots.txt files, implement rate limiting to avoid overwhelming servers, and ensure you're not violating any terms of service.
Python: The Go-To Language for Web Scraping
Python has long been the preferred language for web scraping due to its simplicity and powerful libraries. Libraries like Beautiful Soup and Scrapy have made it easier than ever to extract data from web pages. Here's a basic example of how you might use Python with the requests and Beautiful Soup libraries to scrape a website:
import requestsfrom bs4 import BeautifulSoupurl = 'https://example.com'response = requests.get(url)soup = BeautifulSoup(response.text, 'html.parser')# Extract all paragraph textsparagraphs = soup.find_all('p')for p in paragraphs: print(p.text)
Python's popularity in the data science and machine learning communities also makes it an excellent choice for projects that involve analyzing the scraped data. With libraries like pandas and numpy, you can easily process and visualize the information you've collected.
JavaScript: Scraping in the Browser and Beyond
JavaScript has become increasingly popular for web scraping, especially with the rise of Node.js for server-side scripting. One of the main advantages of using JavaScript is its ability to interact with dynamic web pages that load content asynchronously. Libraries like Puppeteer and Cheerio have made it possible to automate browser interactions and parse HTML with ease. Here's a simple example using Node.js and Cheerio:
const axios = require('axios');const cheerio = require('cheerio');async function scrapeWebsite() { const url = 'https://example.com'; const response = await axios.get(url); const $ = cheerio.load(response.data); // Extract all heading texts $('h1, h2, h3').each((index, element) => { console.log($(element).text()); });}scrapeWebsite();
JavaScript's asynchronous nature makes it well-suited for handling multiple requests concurrently, which can significantly speed up large-scale scraping operations. Additionally, its widespread use in web development means that many developers are already familiar with the language, making it a natural choice for web scraping projects.
Ruby: Elegant Scraping with Nokogiri
Ruby, known for its elegant syntax and developer-friendly ecosystem, offers powerful web scraping capabilities through libraries like Nokogiri. Ruby's simplicity and readability make it an excellent choice for those who prioritize clean, maintainable code. Here's a brief example of web scraping using Ruby and Nokogiri:
require 'nokogiri'require 'open-uri'url = 'https://example.com'doc = Nokogiri::HTML(URI.open(url))# Extract all link texts and URLsdoc.css('a').each do |link| puts "#{link.text} - #{link['href']}"end
Ruby's extensive gem ecosystem provides additional tools for handling various aspects of web scraping, such as handling cookies, managing sessions, and dealing with JavaScript-rendered content. The language's focus on developer happiness also makes it a joy to work with for long-term scraping projects.
Overcoming Common Web Scraping Challenges
Regardless of the programming language you choose, you're likely to encounter some common challenges when scraping websites. Here are some tips to help you navigate these obstacles:
Handling dynamic content: Many modern websites use JavaScript to load content dynamically. To scrape these sites effectively, you may need to use headless browsers or tools that can execute JavaScript, such as Selenium or Puppeteer.
Dealing with rate limiting and IP blocks: Websites often implement measures to prevent excessive scraping. To avoid being blocked, consider using proxy servers, implementing delays between requests, and rotating user agents. At Evomi, we offer reliable proxy solutions that can help you overcome these challenges while ensuring compliance with ethical scraping practices.
Parsing complex HTML structures: Some websites have intricate HTML structures that can be difficult to navigate. Familiarize yourself with advanced CSS selectors and XPath queries to extract data from these complex layouts more effectively.
Handling authentication and sessions: For websites that require login, you'll need to manage cookies and maintain session state. Most programming languages have libraries or modules to help with this, such as the requests-html library for Python.
Scaling your scraping operations: As your data collection needs grow, you may need to scale your scraping operations. Consider using distributed systems, message queues, or cloud-based solutions to handle large-scale scraping tasks efficiently.
Ethical Considerations and Best Practices
While web scraping can be an incredibly powerful tool for data collection, it's crucial to approach it ethically and responsibly. Here are some best practices to keep in mind:
Respect robots.txt: Always check and adhere to the rules specified in a website's robots.txt file. This file outlines which parts of the site can be crawled and at what frequency.
Implement rate limiting: Avoid overwhelming servers by limiting the rate of your requests. This not only helps maintain good relationships with website owners but also reduces the likelihood of your IP being blocked.
Identify your scraper: Use a custom user agent that identifies your scraper and provides contact information. This allows website owners to reach out if they have concerns about your scraping activities.
Be mindful of copyright: Ensure that you're not violating copyright laws when scraping and using content from websites. Some data may be protected, and it's essential to understand the legal implications of your scraping activities.
Consider API alternatives: Before scraping a website, check if they offer an API for data access. Using an official API is often more reliable and respectful than scraping.
At Evomi, we understand the importance of ethical web scraping and provide solutions that help businesses collect data responsibly. Our proxy services are designed to support legitimate data collection efforts while maintaining compliance with best practices and legal requirements.
Conclusion: Choosing the Right Approach for Your Project
Mastering automated web scraping is a valuable skill that can significantly enhance your data collection capabilities. Whether you choose Python, JavaScript, Ruby, or any other programming language, the key is to understand the fundamental concepts and challenges involved in web scraping.
When selecting a language and approach for your web scraping project, consider factors such as your team's expertise, the complexity of the websites you'll be scraping, and the scale of your data collection needs. Remember that the most effective web scraping solutions often combine multiple tools and techniques to overcome various challenges.
As you embark on your web scraping journey, don't hesitate to explore the various libraries and frameworks available in your chosen language. Experiment with different approaches, and always prioritize ethical scraping practices. With the right tools and mindset, you'll be well-equipped to tackle even the most complex web scraping projects and unlock valuable insights from the vast sea of online data.
If you're looking for reliable proxy solutions to support your web scraping efforts, consider giving Evomi a try. Our range of residential, mobile, and datacenter proxies can help you overcome common scraping obstacles while ensuring high-quality, ethical data collection. With competitive pricing starting at just $0.35 per GB for datacenter proxies and $2.15 per GB for residential and mobile proxies, we offer cost-effective solutions for businesses of all sizes. Plus, our free trial allows you to experience the benefits of our services risk-free. Take your web scraping capabilities to the next level with Evomi's robust proxy infrastructure.

Read More Blogs
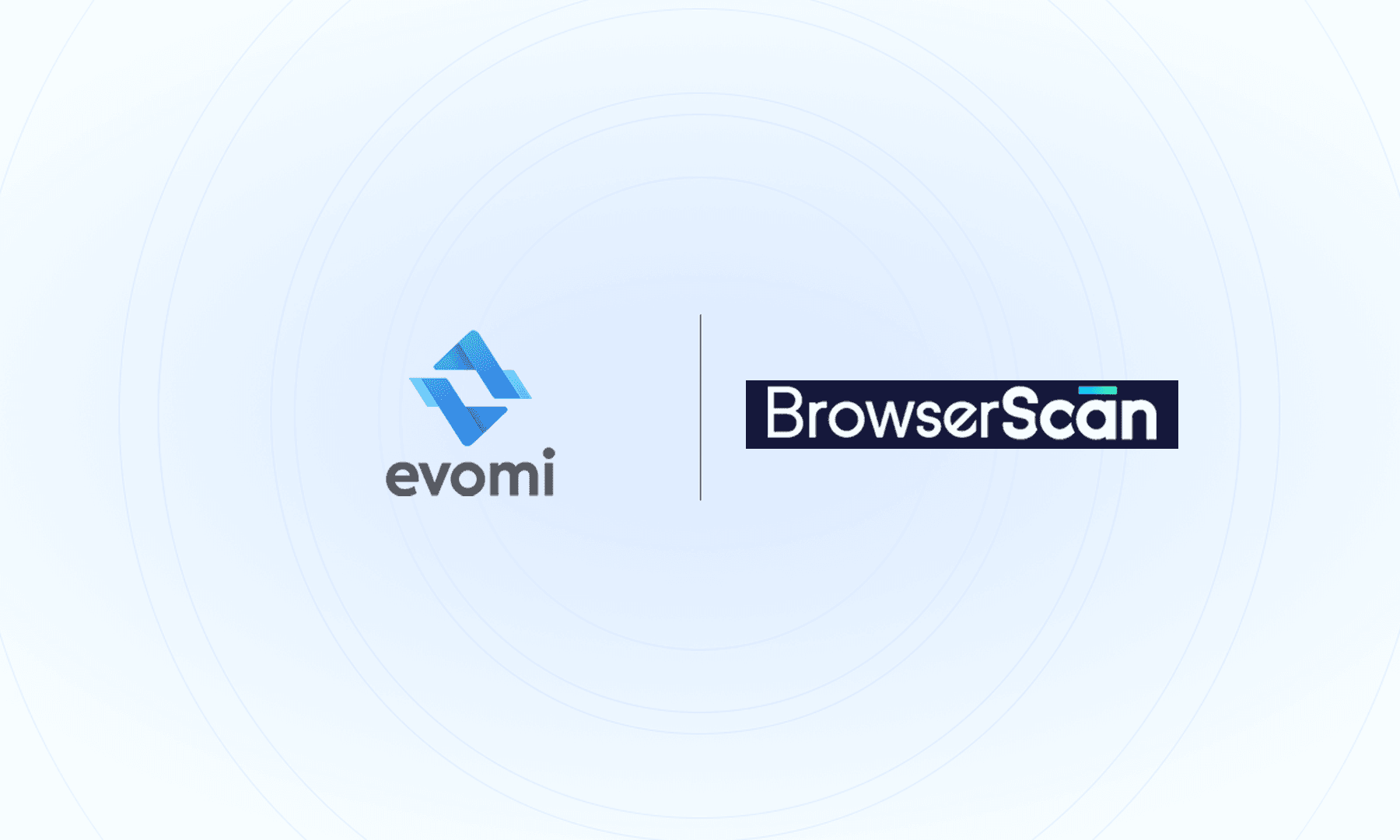
Oct 22, 2024
3 min Read
How to Use BrowserScan to Verify Your Browser Fingerprint
BrowserScan is an advanced tool that analyzes your browser's fingerprints to give you a fingerprint authenticity score. This score is pivotal as a figure below 90% flags potential privacy risks, indicating you may be divulging traces that could be exploited to track your online movements or link your multiple accounts, leading to possible account suspensions.
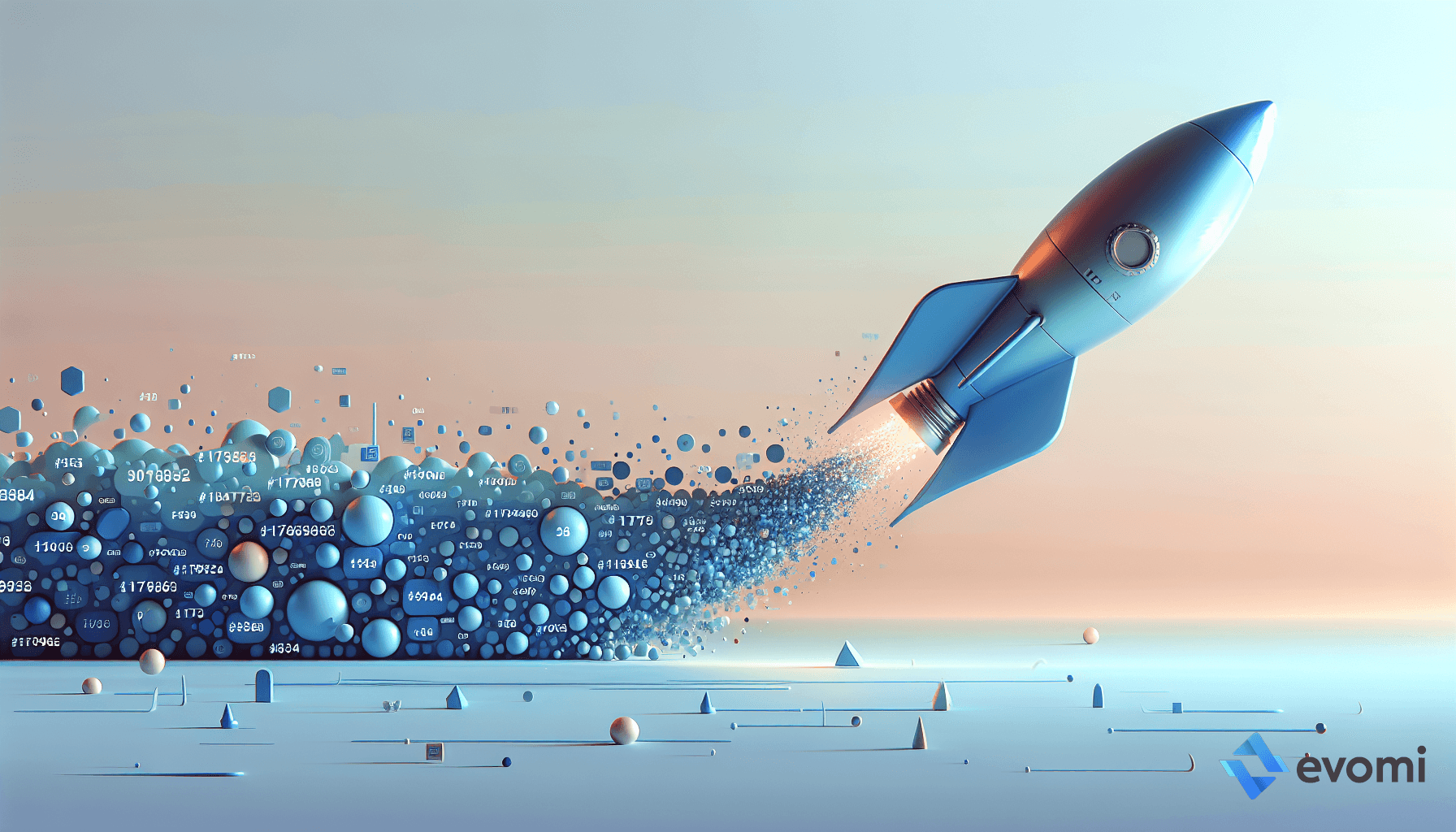
Aug 7, 2024
3 min Read
Boost Web Scraping with Advanced C++ Libraries
Tired of sluggish web scraping performance? Unlock the full potential of your data extraction projects with cutting-edge C++ libraries. Discover how to turbocharge your backend processing and leave Python in the dust.
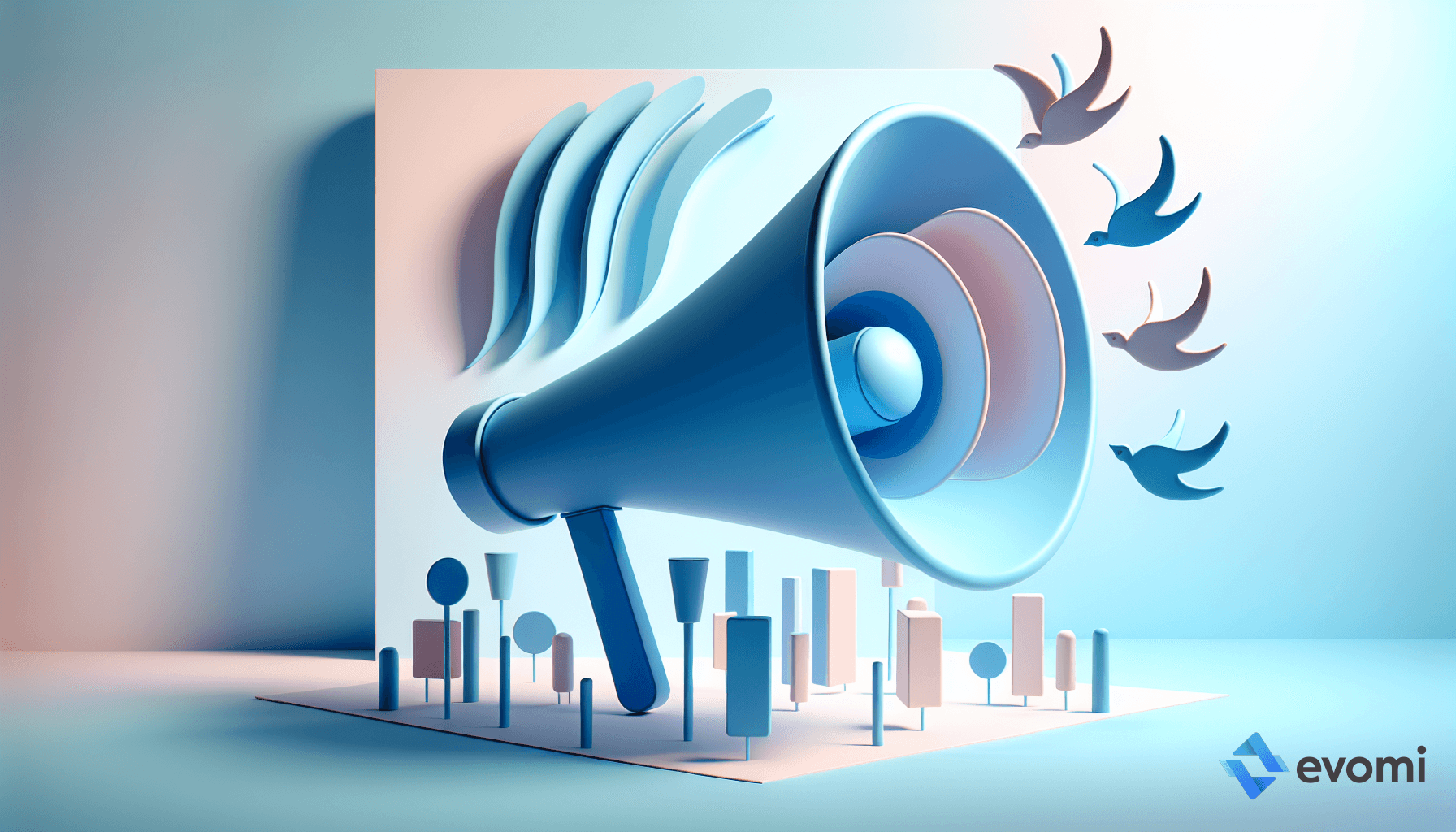
Jun 13, 2024
2 min Read
Amplify Online Influence with Twitter Proxies
Ever wondered how some Twitter users seem to have an uncanny ability to reach vast audiences and dominate conversations? The secret might be simpler than you think. Discover how proxies can be your hidden weapon in the battle for online influence, transforming your Twitter presence from ordinary to extraordinary.
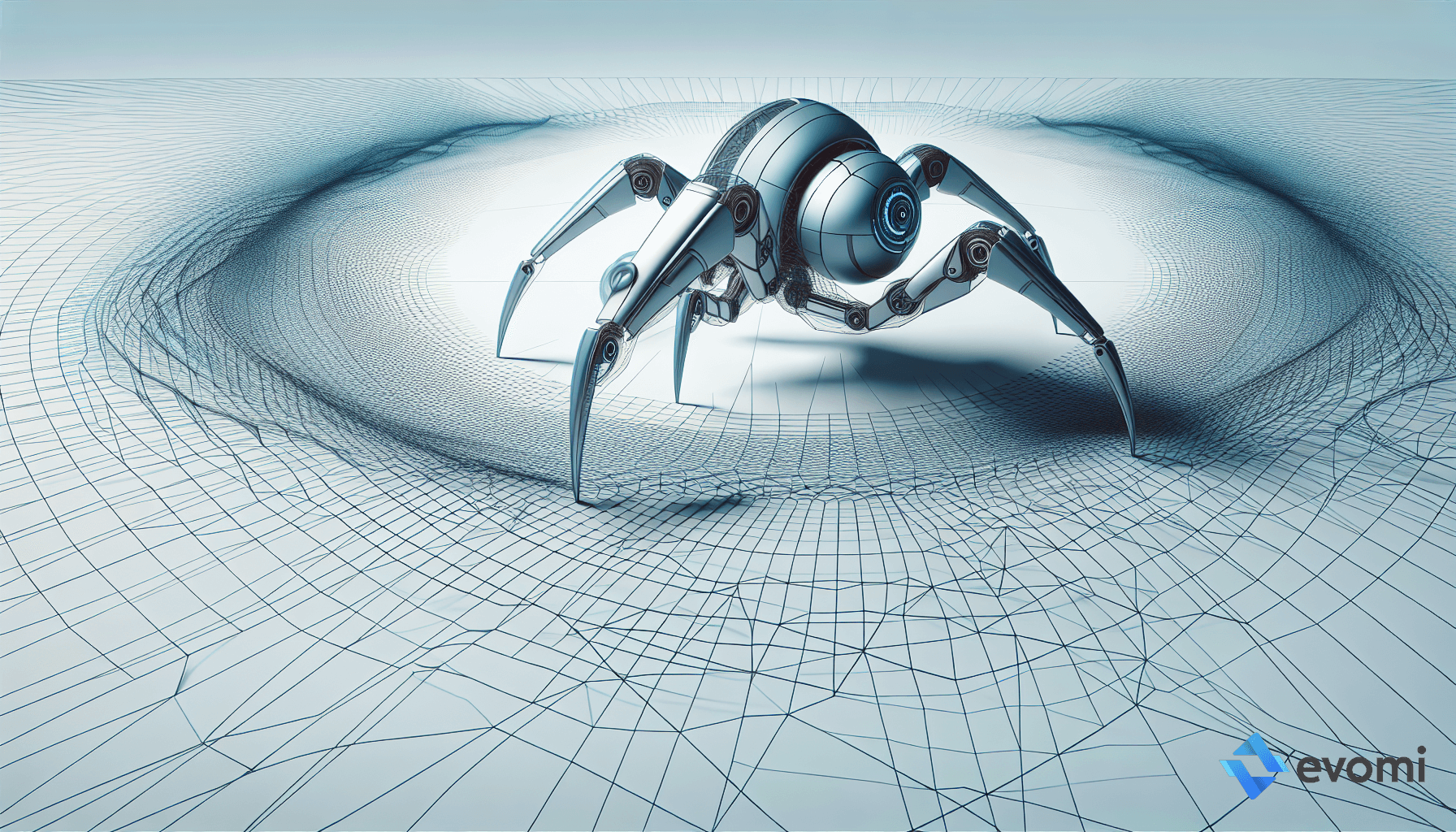
May 29, 2024
3 min Read
10 Stealth Techniques for Unnoticed Web Scraping
Ever feel like a digital ninja, silently gathering data from the vast expanse of the internet? Mastering the art of web scraping without detection is a valuable skill in today's data-driven world. Discover 10 cutting-edge techniques that will help you navigate the web unnoticed, extracting the information you need while staying under the radar.
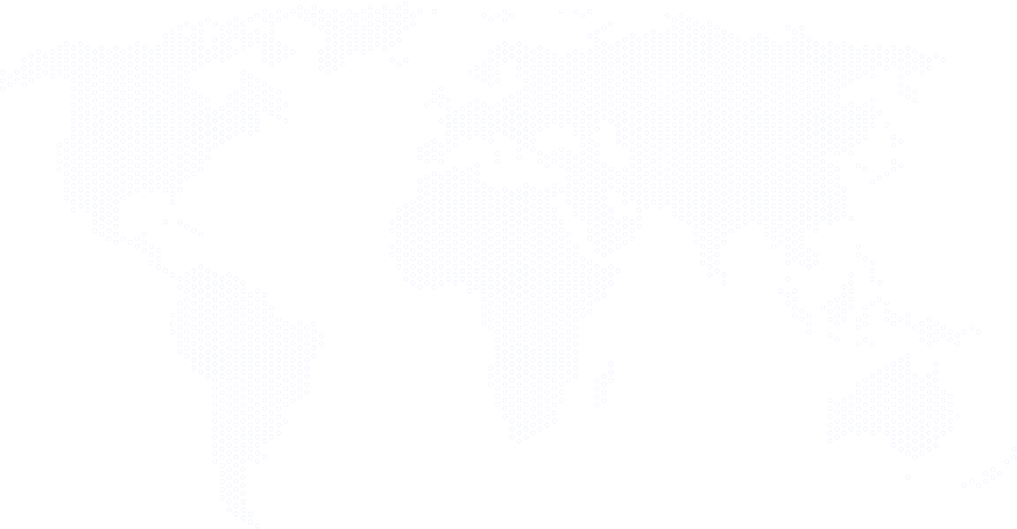
Get in Touch
Products
Get in Touch
Products
Get in Touch
Products