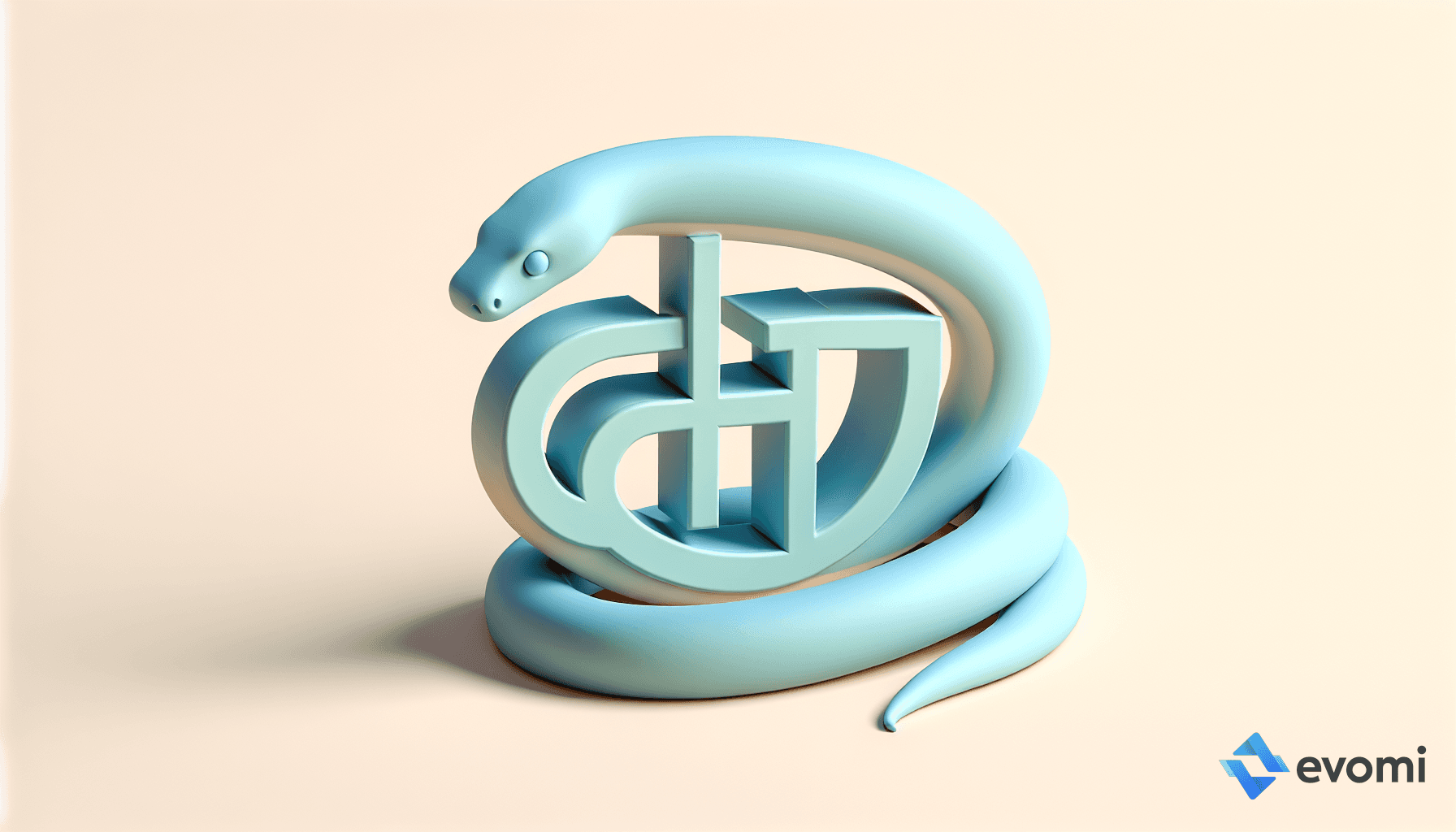
Mastering Python Requests: The Ultimate 2024 Guide for HTTP Communication
In the ever-evolving landscape of web development and data science, mastering HTTP communication is crucial for any Python developer. The Requests library has long been the go-to tool for handling HTTP requests in Python, and in 2024, it remains an indispensable part of the Python ecosystem. This comprehensive guide will take you through the ins and outs of using Requests, from basic GET requests to advanced techniques that will supercharge your web scraping and API interactions.
1. Getting Started with Requests
Before diving into the more complex aspects of Requests, it's essential to understand the basics. The Requests library simplifies HTTP communication by abstracting away many of the complexities involved in making network requests. To get started, you'll need to install Requests using pip:
pip install requests
Once installed, you can import the library and make your first request:
import requestsresponse = requests.get('https://api.example.com/data')print(response.status_code)print(response.text)
This simple example demonstrates how to make a GET request to a URL and print the status code and response content. The beauty of Requests lies in its simplicity – with just a few lines of code, you can interact with web services and APIs effortlessly.
2. Understanding HTTP Methods with Requests
HTTP defines several methods for interacting with web resources, and Requests supports all of them. While GET is the most common, understanding how to use POST, PUT, DELETE, and other methods is crucial for full-fledged API interactions. Let's explore how to use these methods with Requests:
# POST requestresponse = requests.post('https://api.example.com/submit', data={'key': 'value'})# PUT requestresponse = requests.put('https://api.example.com/update', data={'id': 1, 'name': 'Updated Name'})# DELETE requestresponse = requests.delete('https://api.example.com/delete/1')
Each method serves a specific purpose in RESTful API design. POST is typically used for creating new resources, PUT for updating existing ones, and DELETE for removing resources. By mastering these methods, you'll be able to interact with any API effectively, whether you're building a web application, automating tasks, or collecting data for analysis.
3. Handling Authentication and Headers
Many APIs require authentication to access their resources. Requests makes it easy to include authentication credentials and custom headers in your requests. Here's how you can handle different types of authentication:
# Basic Authenticationresponse = requests.get('https://api.example.com/secure', auth=('username', 'password'))# Custom Headersheaders = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}response = requests.get('https://api.example.com/data', headers=headers)# Session-based Authenticationsession = requests.Session()session.auth = ('username', 'password')response = session.get('https://api.example.com/dashboard')
Using sessions can be particularly efficient when making multiple requests to the same host, as it allows you to persist certain parameters across requests. This is especially useful when dealing with APIs that use cookies or other session-based authentication mechanisms.
4. Optimizing Performance with Concurrent Requests
When dealing with large-scale data collection or API interactions, making requests sequentially can be time-consuming. Fortunately, Requests can be combined with Python's asyncio library or third-party libraries like grequests to perform concurrent requests. This can significantly speed up your data gathering processes:
import grequestsurls = [ 'https://api.example.com/data1', 'https://api.example.com/data2', 'https://api.example.com/data3']# Create a set of unsent Requestsreqs = (grequests.get(u) for u in urls)# Send them all at the same timeresponses = grequests.map(reqs)for response in responses: print(response.status_code)
This approach allows you to make multiple requests simultaneously, drastically reducing the time required to fetch data from multiple endpoints. However, it's important to use this technique responsibly and in accordance with the API's rate limiting policies to avoid overwhelming the server or getting your requests blocked.
5. Error Handling and Retries
Network requests can fail for various reasons, from server errors to network interruptions. Robust error handling is crucial for building reliable applications that interact with web services. Requests provides several ways to handle errors and implement retry logic:
from requests.exceptions import RequestExceptionfrom time import sleepdef make_request_with_retry(url, max_retries=3): for attempt in range(max_retries): try: response = requests.get(url) response.raise_for_status() # Raises an HTTPError for bad responses return response except RequestException as e: print(f"Request failed: {e}") if attempt < max_retries - 1: sleep_time = 2 ** attempt # Exponential backoff print(f"Retrying in {sleep_time} seconds...") sleep(sleep_time) else: print("Max retries reached. Giving up.") raise# Usagetry: response = make_request_with_retry('https://api.example.com/data') print(response.json())except RequestException as e: print(f"Failed to fetch data: {e}")
This example demonstrates how to implement a retry mechanism with exponential backoff, which is a best practice for handling transient errors. By catching specific exceptions and implementing smart retry logic, you can create more resilient applications that gracefully handle network issues.
6. Leveraging Proxies for Advanced Use Cases
In some scenarios, you may need to route your requests through a proxy server. This could be for various reasons, such as accessing geo-restricted content, load balancing, or maintaining anonymity. Requests makes it easy to use proxies:
proxies = { 'http': 'http://10.10.1.10:3128', 'https': 'http://10.10.1.10:1080',}response = requests.get('http://example.org', proxies=proxies)
For more advanced proxy usage, especially in business contexts where data intelligence and web scraping are crucial, specialized proxy services can be invaluable. At Evomi, we offer high-quality residential, mobile, and datacenter proxies that can significantly enhance your data collection capabilities. Our proxies are designed to handle high-volume requests while maintaining reliability and performance, making them ideal for businesses engaged in market research, competitor analysis, or large-scale data gathering.
Conclusion
Mastering the Requests library is an essential skill for any Python developer working with web services or APIs. From basic GET requests to advanced techniques like concurrent requests and error handling, Requests provides a powerful and flexible toolkit for HTTP communication. As you continue to develop your skills, remember that the key to successful web scraping and API interaction lies not just in the code you write, but also in the infrastructure you use.
For those looking to take their data collection and API interactions to the next level, consider exploring Evomi's proxy solutions. Our Swiss-based service offers high-quality, cost-effective proxies that can help you overcome common challenges in web scraping and data intelligence. With plans starting at just $0.35 per GB for datacenter proxies and $2.15 per GB for residential and mobile proxies, we provide an accessible solution for businesses of all sizes.
Remember, whether you're building a simple script to fetch data from an API or developing a complex web scraping system, the principles covered in this guide will serve as a solid foundation. Keep experimenting, stay curious, and don't hesitate to leverage advanced tools and services to achieve your data collection goals.
Mastering Python Requests: The Ultimate 2024 Guide for HTTP Communication
In the ever-evolving landscape of web development and data science, mastering HTTP communication is crucial for any Python developer. The Requests library has long been the go-to tool for handling HTTP requests in Python, and in 2024, it remains an indispensable part of the Python ecosystem. This comprehensive guide will take you through the ins and outs of using Requests, from basic GET requests to advanced techniques that will supercharge your web scraping and API interactions.
1. Getting Started with Requests
Before diving into the more complex aspects of Requests, it's essential to understand the basics. The Requests library simplifies HTTP communication by abstracting away many of the complexities involved in making network requests. To get started, you'll need to install Requests using pip:
pip install requests
Once installed, you can import the library and make your first request:
import requestsresponse = requests.get('https://api.example.com/data')print(response.status_code)print(response.text)
This simple example demonstrates how to make a GET request to a URL and print the status code and response content. The beauty of Requests lies in its simplicity – with just a few lines of code, you can interact with web services and APIs effortlessly.
2. Understanding HTTP Methods with Requests
HTTP defines several methods for interacting with web resources, and Requests supports all of them. While GET is the most common, understanding how to use POST, PUT, DELETE, and other methods is crucial for full-fledged API interactions. Let's explore how to use these methods with Requests:
# POST requestresponse = requests.post('https://api.example.com/submit', data={'key': 'value'})# PUT requestresponse = requests.put('https://api.example.com/update', data={'id': 1, 'name': 'Updated Name'})# DELETE requestresponse = requests.delete('https://api.example.com/delete/1')
Each method serves a specific purpose in RESTful API design. POST is typically used for creating new resources, PUT for updating existing ones, and DELETE for removing resources. By mastering these methods, you'll be able to interact with any API effectively, whether you're building a web application, automating tasks, or collecting data for analysis.
3. Handling Authentication and Headers
Many APIs require authentication to access their resources. Requests makes it easy to include authentication credentials and custom headers in your requests. Here's how you can handle different types of authentication:
# Basic Authenticationresponse = requests.get('https://api.example.com/secure', auth=('username', 'password'))# Custom Headersheaders = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}response = requests.get('https://api.example.com/data', headers=headers)# Session-based Authenticationsession = requests.Session()session.auth = ('username', 'password')response = session.get('https://api.example.com/dashboard')
Using sessions can be particularly efficient when making multiple requests to the same host, as it allows you to persist certain parameters across requests. This is especially useful when dealing with APIs that use cookies or other session-based authentication mechanisms.
4. Optimizing Performance with Concurrent Requests
When dealing with large-scale data collection or API interactions, making requests sequentially can be time-consuming. Fortunately, Requests can be combined with Python's asyncio library or third-party libraries like grequests to perform concurrent requests. This can significantly speed up your data gathering processes:
import grequestsurls = [ 'https://api.example.com/data1', 'https://api.example.com/data2', 'https://api.example.com/data3']# Create a set of unsent Requestsreqs = (grequests.get(u) for u in urls)# Send them all at the same timeresponses = grequests.map(reqs)for response in responses: print(response.status_code)
This approach allows you to make multiple requests simultaneously, drastically reducing the time required to fetch data from multiple endpoints. However, it's important to use this technique responsibly and in accordance with the API's rate limiting policies to avoid overwhelming the server or getting your requests blocked.
5. Error Handling and Retries
Network requests can fail for various reasons, from server errors to network interruptions. Robust error handling is crucial for building reliable applications that interact with web services. Requests provides several ways to handle errors and implement retry logic:
from requests.exceptions import RequestExceptionfrom time import sleepdef make_request_with_retry(url, max_retries=3): for attempt in range(max_retries): try: response = requests.get(url) response.raise_for_status() # Raises an HTTPError for bad responses return response except RequestException as e: print(f"Request failed: {e}") if attempt < max_retries - 1: sleep_time = 2 ** attempt # Exponential backoff print(f"Retrying in {sleep_time} seconds...") sleep(sleep_time) else: print("Max retries reached. Giving up.") raise# Usagetry: response = make_request_with_retry('https://api.example.com/data') print(response.json())except RequestException as e: print(f"Failed to fetch data: {e}")
This example demonstrates how to implement a retry mechanism with exponential backoff, which is a best practice for handling transient errors. By catching specific exceptions and implementing smart retry logic, you can create more resilient applications that gracefully handle network issues.
6. Leveraging Proxies for Advanced Use Cases
In some scenarios, you may need to route your requests through a proxy server. This could be for various reasons, such as accessing geo-restricted content, load balancing, or maintaining anonymity. Requests makes it easy to use proxies:
proxies = { 'http': 'http://10.10.1.10:3128', 'https': 'http://10.10.1.10:1080',}response = requests.get('http://example.org', proxies=proxies)
For more advanced proxy usage, especially in business contexts where data intelligence and web scraping are crucial, specialized proxy services can be invaluable. At Evomi, we offer high-quality residential, mobile, and datacenter proxies that can significantly enhance your data collection capabilities. Our proxies are designed to handle high-volume requests while maintaining reliability and performance, making them ideal for businesses engaged in market research, competitor analysis, or large-scale data gathering.
Conclusion
Mastering the Requests library is an essential skill for any Python developer working with web services or APIs. From basic GET requests to advanced techniques like concurrent requests and error handling, Requests provides a powerful and flexible toolkit for HTTP communication. As you continue to develop your skills, remember that the key to successful web scraping and API interaction lies not just in the code you write, but also in the infrastructure you use.
For those looking to take their data collection and API interactions to the next level, consider exploring Evomi's proxy solutions. Our Swiss-based service offers high-quality, cost-effective proxies that can help you overcome common challenges in web scraping and data intelligence. With plans starting at just $0.35 per GB for datacenter proxies and $2.15 per GB for residential and mobile proxies, we provide an accessible solution for businesses of all sizes.
Remember, whether you're building a simple script to fetch data from an API or developing a complex web scraping system, the principles covered in this guide will serve as a solid foundation. Keep experimenting, stay curious, and don't hesitate to leverage advanced tools and services to achieve your data collection goals.
Mastering Python Requests: The Ultimate 2024 Guide for HTTP Communication
In the ever-evolving landscape of web development and data science, mastering HTTP communication is crucial for any Python developer. The Requests library has long been the go-to tool for handling HTTP requests in Python, and in 2024, it remains an indispensable part of the Python ecosystem. This comprehensive guide will take you through the ins and outs of using Requests, from basic GET requests to advanced techniques that will supercharge your web scraping and API interactions.
1. Getting Started with Requests
Before diving into the more complex aspects of Requests, it's essential to understand the basics. The Requests library simplifies HTTP communication by abstracting away many of the complexities involved in making network requests. To get started, you'll need to install Requests using pip:
pip install requests
Once installed, you can import the library and make your first request:
import requestsresponse = requests.get('https://api.example.com/data')print(response.status_code)print(response.text)
This simple example demonstrates how to make a GET request to a URL and print the status code and response content. The beauty of Requests lies in its simplicity – with just a few lines of code, you can interact with web services and APIs effortlessly.
2. Understanding HTTP Methods with Requests
HTTP defines several methods for interacting with web resources, and Requests supports all of them. While GET is the most common, understanding how to use POST, PUT, DELETE, and other methods is crucial for full-fledged API interactions. Let's explore how to use these methods with Requests:
# POST requestresponse = requests.post('https://api.example.com/submit', data={'key': 'value'})# PUT requestresponse = requests.put('https://api.example.com/update', data={'id': 1, 'name': 'Updated Name'})# DELETE requestresponse = requests.delete('https://api.example.com/delete/1')
Each method serves a specific purpose in RESTful API design. POST is typically used for creating new resources, PUT for updating existing ones, and DELETE for removing resources. By mastering these methods, you'll be able to interact with any API effectively, whether you're building a web application, automating tasks, or collecting data for analysis.
3. Handling Authentication and Headers
Many APIs require authentication to access their resources. Requests makes it easy to include authentication credentials and custom headers in your requests. Here's how you can handle different types of authentication:
# Basic Authenticationresponse = requests.get('https://api.example.com/secure', auth=('username', 'password'))# Custom Headersheaders = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}response = requests.get('https://api.example.com/data', headers=headers)# Session-based Authenticationsession = requests.Session()session.auth = ('username', 'password')response = session.get('https://api.example.com/dashboard')
Using sessions can be particularly efficient when making multiple requests to the same host, as it allows you to persist certain parameters across requests. This is especially useful when dealing with APIs that use cookies or other session-based authentication mechanisms.
4. Optimizing Performance with Concurrent Requests
When dealing with large-scale data collection or API interactions, making requests sequentially can be time-consuming. Fortunately, Requests can be combined with Python's asyncio library or third-party libraries like grequests to perform concurrent requests. This can significantly speed up your data gathering processes:
import grequestsurls = [ 'https://api.example.com/data1', 'https://api.example.com/data2', 'https://api.example.com/data3']# Create a set of unsent Requestsreqs = (grequests.get(u) for u in urls)# Send them all at the same timeresponses = grequests.map(reqs)for response in responses: print(response.status_code)
This approach allows you to make multiple requests simultaneously, drastically reducing the time required to fetch data from multiple endpoints. However, it's important to use this technique responsibly and in accordance with the API's rate limiting policies to avoid overwhelming the server or getting your requests blocked.
5. Error Handling and Retries
Network requests can fail for various reasons, from server errors to network interruptions. Robust error handling is crucial for building reliable applications that interact with web services. Requests provides several ways to handle errors and implement retry logic:
from requests.exceptions import RequestExceptionfrom time import sleepdef make_request_with_retry(url, max_retries=3): for attempt in range(max_retries): try: response = requests.get(url) response.raise_for_status() # Raises an HTTPError for bad responses return response except RequestException as e: print(f"Request failed: {e}") if attempt < max_retries - 1: sleep_time = 2 ** attempt # Exponential backoff print(f"Retrying in {sleep_time} seconds...") sleep(sleep_time) else: print("Max retries reached. Giving up.") raise# Usagetry: response = make_request_with_retry('https://api.example.com/data') print(response.json())except RequestException as e: print(f"Failed to fetch data: {e}")
This example demonstrates how to implement a retry mechanism with exponential backoff, which is a best practice for handling transient errors. By catching specific exceptions and implementing smart retry logic, you can create more resilient applications that gracefully handle network issues.
6. Leveraging Proxies for Advanced Use Cases
In some scenarios, you may need to route your requests through a proxy server. This could be for various reasons, such as accessing geo-restricted content, load balancing, or maintaining anonymity. Requests makes it easy to use proxies:
proxies = { 'http': 'http://10.10.1.10:3128', 'https': 'http://10.10.1.10:1080',}response = requests.get('http://example.org', proxies=proxies)
For more advanced proxy usage, especially in business contexts where data intelligence and web scraping are crucial, specialized proxy services can be invaluable. At Evomi, we offer high-quality residential, mobile, and datacenter proxies that can significantly enhance your data collection capabilities. Our proxies are designed to handle high-volume requests while maintaining reliability and performance, making them ideal for businesses engaged in market research, competitor analysis, or large-scale data gathering.
Conclusion
Mastering the Requests library is an essential skill for any Python developer working with web services or APIs. From basic GET requests to advanced techniques like concurrent requests and error handling, Requests provides a powerful and flexible toolkit for HTTP communication. As you continue to develop your skills, remember that the key to successful web scraping and API interaction lies not just in the code you write, but also in the infrastructure you use.
For those looking to take their data collection and API interactions to the next level, consider exploring Evomi's proxy solutions. Our Swiss-based service offers high-quality, cost-effective proxies that can help you overcome common challenges in web scraping and data intelligence. With plans starting at just $0.35 per GB for datacenter proxies and $2.15 per GB for residential and mobile proxies, we provide an accessible solution for businesses of all sizes.
Remember, whether you're building a simple script to fetch data from an API or developing a complex web scraping system, the principles covered in this guide will serve as a solid foundation. Keep experimenting, stay curious, and don't hesitate to leverage advanced tools and services to achieve your data collection goals.
