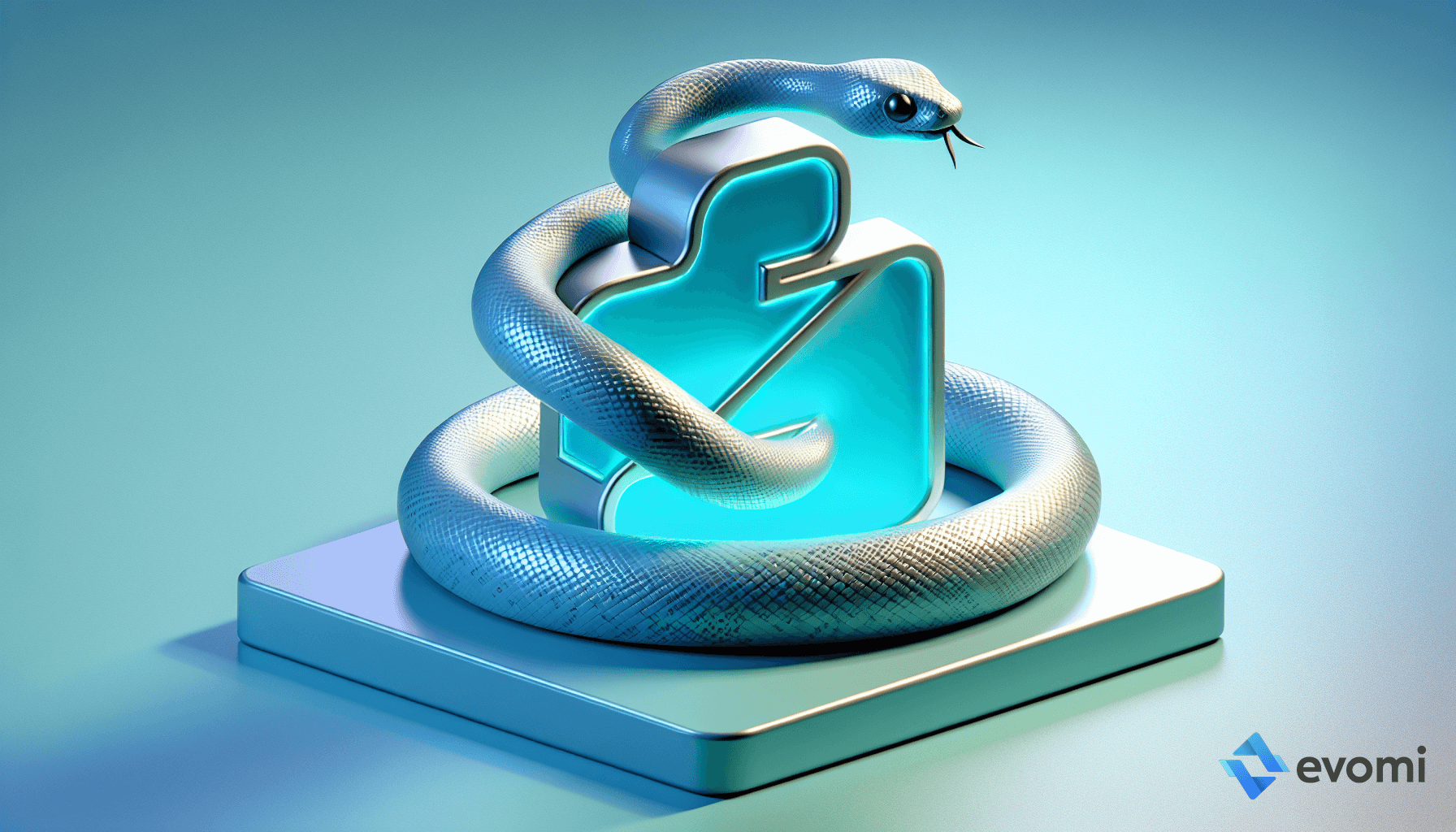
Mastering JSON Manipulation in Python: A Guide to Parsing, Reading, and Writing
JSON (JavaScript Object Notation) has become the go-to format for data exchange in modern web applications and APIs. As a Python developer, understanding how to work with JSON is crucial for handling data effectively. In this guide, we'll dive deep into the world of JSON manipulation in Python, covering everything from parsing and reading to writing and modifying JSON data. Whether you're building web scraping tools, working with APIs, or developing data-driven applications, these skills will prove invaluable in your coding journey.
Understanding JSON: The Basics
Before we dive into the nitty-gritty of JSON manipulation in Python, let's take a moment to understand what JSON is and why it's so popular. JSON is a lightweight, text-based data interchange format that's easy for humans to read and write, and easy for machines to parse and generate. It's built on two main structures: objects (represented as key-value pairs) and arrays (ordered lists of values). These simple yet powerful structures make JSON an ideal choice for storing and transmitting structured data.
In Python, JSON data is typically represented as dictionaries and lists, making it a natural fit for the language's data structures. This compatibility is one of the reasons why Python is such a popular choice for working with JSON data, especially in data analysis and web development projects.
Parsing JSON in Python: From Strings to Objects
The first step in working with JSON data in Python is parsing it. The built-in json
module provides all the tools we need to convert JSON strings into Python objects. Let's look at a simple example:
import jsonjson_string = '{"name": "John Doe", "age": 30, "city": "New York"}'parsed_data = json.loads(json_string)print(parsed_data['name']) # Output: John Doeprint(type(parsed_data)) # Output: <class 'dict'>
In this example, we use the json.loads()
function to parse a JSON string into a Python dictionary. The resulting object can be accessed just like any other dictionary in Python. This method is particularly useful when working with API responses or reading JSON data from files.
For more complex JSON structures, Python's ability to nest dictionaries and lists comes in handy. You can easily navigate through nested JSON data using square bracket notation or the get()
method for dictionaries:
complex_json = '''{ "person": { "name": "Jane Smith", "age": 28, "address": { "street": "123 Main St", "city": "Boston" }, "hobbies": ["reading", "hiking", "photography"] }}'''data = json.loads(complex_json)print(data['person']['address']['city']) # Output: Bostonprint(data['person']['hobbies'][1]) # Output: hiking
Reading JSON from Files: Handling External Data
While parsing JSON strings is useful, you'll often find yourself working with JSON data stored in files. Python makes this process straightforward with the json.load()
function. Here's how you can read JSON data from a file:
with open('data.json', 'r') as file: data = json.load(file)print(data)
This method is particularly useful when working with large datasets or configuration files. It allows you to easily import and work with JSON data stored on your local machine or server. When dealing with large JSON files, it's important to consider memory usage. For extremely large files, you might want to consider streaming the JSON data or using libraries like ijson
for iterative parsing.
Writing JSON: Serializing Python Objects
Creating JSON data from Python objects is just as important as parsing it. The json.dumps()
function allows you to convert Python objects into JSON strings, while json.dump()
writes JSON data directly to a file. Let's look at both methods:
# Converting a Python object to a JSON stringpython_dict = { "name": "Alice", "age": 25, "is_student": False, "grades": [85, 90, 88]}json_string = json.dumps(python_dict, indent=2)print(json_string)# Writing JSON data to a filewith open('output.json', 'w') as file: json.dump(python_dict, file, indent=2)
The indent
parameter is optional but useful for creating human-readable JSON output. It specifies the number of spaces to use for indentation. When working with more complex data structures or custom objects, you might need to use the default
parameter of json.dumps()
to specify how to serialize objects that aren't natively supported by JSON.
Advanced JSON Manipulation: Tips and Tricks
As you become more comfortable with basic JSON operations in Python, you'll want to explore some advanced techniques to make your code more efficient and powerful. Here are a few tips and tricks to take your JSON manipulation skills to the next level:
Use the
default
parameter for custom serialization: When working with custom objects, you can define a function to specify how they should be serialized to JSON.Leverage
json.tool
for pretty-printing: Python'sjson.tool
module provides a command-line interface for formatting JSON data, which can be useful for debugging or presenting data.Explore alternative libraries: While the built-in
json
module is sufficient for most tasks, libraries likesimplejson
orujson
can offer performance improvements for large-scale applications.Handle encoding issues: When working with JSON data that contains non-ASCII characters, be sure to specify the correct encoding to avoid errors.
Use JSON schema validation: For applications that require strict data validation, consider using JSON schema libraries to ensure your data conforms to a predefined structure.
Practical Applications: JSON in the Real World
Understanding JSON manipulation in Python opens up a world of possibilities for data-driven applications. At Evomi, we've seen firsthand how crucial these skills are for businesses looking to leverage data intelligence and web scraping. Our clients use Python and JSON manipulation for a variety of purposes, including:
Extracting structured data from web APIs for market research
Parsing and analyzing large datasets for business intelligence
Building custom data pipelines for SEO optimization
Creating robust configuration systems for scalable applications
By mastering JSON manipulation in Python, you're not just learning a technical skill – you're equipping yourself with the ability to work with the lifeblood of modern data-driven businesses. Whether you're scraping web data, integrating with third-party APIs, or building your own data services, these skills will prove invaluable.
Conclusion: Empowering Your Data Handling Capabilities
JSON manipulation in Python is a fundamental skill for any developer working with data in today's interconnected digital landscape. From parsing API responses to creating data-rich applications, the ability to effortlessly work with JSON will make you a more effective and efficient programmer.
As you continue to explore the world of JSON and Python, remember that practice is key. Try working with different types of JSON data, experiment with more complex structures, and don't be afraid to dive into real-world applications. The more you work with JSON, the more natural and intuitive it will become.
At Evomi, we're committed to helping businesses leverage data intelligence and web scraping technologies to gain a competitive edge. Our range of proxy solutions, including Residential, Mobile, and Datacenter options, are designed to support your data collection and analysis needs. Whether you're just starting out or looking to scale your data operations, we offer flexible plans and a free trial to help you get started.
Remember, the world of data is vast and ever-changing. By mastering JSON manipulation in Python, you're taking a significant step towards becoming a data-savvy developer ready to tackle the challenges of modern software development. Keep learning, keep experimenting, and most importantly, keep building amazing things with your newfound skills!
Mastering JSON Manipulation in Python: A Guide to Parsing, Reading, and Writing
JSON (JavaScript Object Notation) has become the go-to format for data exchange in modern web applications and APIs. As a Python developer, understanding how to work with JSON is crucial for handling data effectively. In this guide, we'll dive deep into the world of JSON manipulation in Python, covering everything from parsing and reading to writing and modifying JSON data. Whether you're building web scraping tools, working with APIs, or developing data-driven applications, these skills will prove invaluable in your coding journey.
Understanding JSON: The Basics
Before we dive into the nitty-gritty of JSON manipulation in Python, let's take a moment to understand what JSON is and why it's so popular. JSON is a lightweight, text-based data interchange format that's easy for humans to read and write, and easy for machines to parse and generate. It's built on two main structures: objects (represented as key-value pairs) and arrays (ordered lists of values). These simple yet powerful structures make JSON an ideal choice for storing and transmitting structured data.
In Python, JSON data is typically represented as dictionaries and lists, making it a natural fit for the language's data structures. This compatibility is one of the reasons why Python is such a popular choice for working with JSON data, especially in data analysis and web development projects.
Parsing JSON in Python: From Strings to Objects
The first step in working with JSON data in Python is parsing it. The built-in json
module provides all the tools we need to convert JSON strings into Python objects. Let's look at a simple example:
import jsonjson_string = '{"name": "John Doe", "age": 30, "city": "New York"}'parsed_data = json.loads(json_string)print(parsed_data['name']) # Output: John Doeprint(type(parsed_data)) # Output: <class 'dict'>
In this example, we use the json.loads()
function to parse a JSON string into a Python dictionary. The resulting object can be accessed just like any other dictionary in Python. This method is particularly useful when working with API responses or reading JSON data from files.
For more complex JSON structures, Python's ability to nest dictionaries and lists comes in handy. You can easily navigate through nested JSON data using square bracket notation or the get()
method for dictionaries:
complex_json = '''{ "person": { "name": "Jane Smith", "age": 28, "address": { "street": "123 Main St", "city": "Boston" }, "hobbies": ["reading", "hiking", "photography"] }}'''data = json.loads(complex_json)print(data['person']['address']['city']) # Output: Bostonprint(data['person']['hobbies'][1]) # Output: hiking
Reading JSON from Files: Handling External Data
While parsing JSON strings is useful, you'll often find yourself working with JSON data stored in files. Python makes this process straightforward with the json.load()
function. Here's how you can read JSON data from a file:
with open('data.json', 'r') as file: data = json.load(file)print(data)
This method is particularly useful when working with large datasets or configuration files. It allows you to easily import and work with JSON data stored on your local machine or server. When dealing with large JSON files, it's important to consider memory usage. For extremely large files, you might want to consider streaming the JSON data or using libraries like ijson
for iterative parsing.
Writing JSON: Serializing Python Objects
Creating JSON data from Python objects is just as important as parsing it. The json.dumps()
function allows you to convert Python objects into JSON strings, while json.dump()
writes JSON data directly to a file. Let's look at both methods:
# Converting a Python object to a JSON stringpython_dict = { "name": "Alice", "age": 25, "is_student": False, "grades": [85, 90, 88]}json_string = json.dumps(python_dict, indent=2)print(json_string)# Writing JSON data to a filewith open('output.json', 'w') as file: json.dump(python_dict, file, indent=2)
The indent
parameter is optional but useful for creating human-readable JSON output. It specifies the number of spaces to use for indentation. When working with more complex data structures or custom objects, you might need to use the default
parameter of json.dumps()
to specify how to serialize objects that aren't natively supported by JSON.
Advanced JSON Manipulation: Tips and Tricks
As you become more comfortable with basic JSON operations in Python, you'll want to explore some advanced techniques to make your code more efficient and powerful. Here are a few tips and tricks to take your JSON manipulation skills to the next level:
Use the
default
parameter for custom serialization: When working with custom objects, you can define a function to specify how they should be serialized to JSON.Leverage
json.tool
for pretty-printing: Python'sjson.tool
module provides a command-line interface for formatting JSON data, which can be useful for debugging or presenting data.Explore alternative libraries: While the built-in
json
module is sufficient for most tasks, libraries likesimplejson
orujson
can offer performance improvements for large-scale applications.Handle encoding issues: When working with JSON data that contains non-ASCII characters, be sure to specify the correct encoding to avoid errors.
Use JSON schema validation: For applications that require strict data validation, consider using JSON schema libraries to ensure your data conforms to a predefined structure.
Practical Applications: JSON in the Real World
Understanding JSON manipulation in Python opens up a world of possibilities for data-driven applications. At Evomi, we've seen firsthand how crucial these skills are for businesses looking to leverage data intelligence and web scraping. Our clients use Python and JSON manipulation for a variety of purposes, including:
Extracting structured data from web APIs for market research
Parsing and analyzing large datasets for business intelligence
Building custom data pipelines for SEO optimization
Creating robust configuration systems for scalable applications
By mastering JSON manipulation in Python, you're not just learning a technical skill – you're equipping yourself with the ability to work with the lifeblood of modern data-driven businesses. Whether you're scraping web data, integrating with third-party APIs, or building your own data services, these skills will prove invaluable.
Conclusion: Empowering Your Data Handling Capabilities
JSON manipulation in Python is a fundamental skill for any developer working with data in today's interconnected digital landscape. From parsing API responses to creating data-rich applications, the ability to effortlessly work with JSON will make you a more effective and efficient programmer.
As you continue to explore the world of JSON and Python, remember that practice is key. Try working with different types of JSON data, experiment with more complex structures, and don't be afraid to dive into real-world applications. The more you work with JSON, the more natural and intuitive it will become.
At Evomi, we're committed to helping businesses leverage data intelligence and web scraping technologies to gain a competitive edge. Our range of proxy solutions, including Residential, Mobile, and Datacenter options, are designed to support your data collection and analysis needs. Whether you're just starting out or looking to scale your data operations, we offer flexible plans and a free trial to help you get started.
Remember, the world of data is vast and ever-changing. By mastering JSON manipulation in Python, you're taking a significant step towards becoming a data-savvy developer ready to tackle the challenges of modern software development. Keep learning, keep experimenting, and most importantly, keep building amazing things with your newfound skills!
Mastering JSON Manipulation in Python: A Guide to Parsing, Reading, and Writing
JSON (JavaScript Object Notation) has become the go-to format for data exchange in modern web applications and APIs. As a Python developer, understanding how to work with JSON is crucial for handling data effectively. In this guide, we'll dive deep into the world of JSON manipulation in Python, covering everything from parsing and reading to writing and modifying JSON data. Whether you're building web scraping tools, working with APIs, or developing data-driven applications, these skills will prove invaluable in your coding journey.
Understanding JSON: The Basics
Before we dive into the nitty-gritty of JSON manipulation in Python, let's take a moment to understand what JSON is and why it's so popular. JSON is a lightweight, text-based data interchange format that's easy for humans to read and write, and easy for machines to parse and generate. It's built on two main structures: objects (represented as key-value pairs) and arrays (ordered lists of values). These simple yet powerful structures make JSON an ideal choice for storing and transmitting structured data.
In Python, JSON data is typically represented as dictionaries and lists, making it a natural fit for the language's data structures. This compatibility is one of the reasons why Python is such a popular choice for working with JSON data, especially in data analysis and web development projects.
Parsing JSON in Python: From Strings to Objects
The first step in working with JSON data in Python is parsing it. The built-in json
module provides all the tools we need to convert JSON strings into Python objects. Let's look at a simple example:
import jsonjson_string = '{"name": "John Doe", "age": 30, "city": "New York"}'parsed_data = json.loads(json_string)print(parsed_data['name']) # Output: John Doeprint(type(parsed_data)) # Output: <class 'dict'>
In this example, we use the json.loads()
function to parse a JSON string into a Python dictionary. The resulting object can be accessed just like any other dictionary in Python. This method is particularly useful when working with API responses or reading JSON data from files.
For more complex JSON structures, Python's ability to nest dictionaries and lists comes in handy. You can easily navigate through nested JSON data using square bracket notation or the get()
method for dictionaries:
complex_json = '''{ "person": { "name": "Jane Smith", "age": 28, "address": { "street": "123 Main St", "city": "Boston" }, "hobbies": ["reading", "hiking", "photography"] }}'''data = json.loads(complex_json)print(data['person']['address']['city']) # Output: Bostonprint(data['person']['hobbies'][1]) # Output: hiking
Reading JSON from Files: Handling External Data
While parsing JSON strings is useful, you'll often find yourself working with JSON data stored in files. Python makes this process straightforward with the json.load()
function. Here's how you can read JSON data from a file:
with open('data.json', 'r') as file: data = json.load(file)print(data)
This method is particularly useful when working with large datasets or configuration files. It allows you to easily import and work with JSON data stored on your local machine or server. When dealing with large JSON files, it's important to consider memory usage. For extremely large files, you might want to consider streaming the JSON data or using libraries like ijson
for iterative parsing.
Writing JSON: Serializing Python Objects
Creating JSON data from Python objects is just as important as parsing it. The json.dumps()
function allows you to convert Python objects into JSON strings, while json.dump()
writes JSON data directly to a file. Let's look at both methods:
# Converting a Python object to a JSON stringpython_dict = { "name": "Alice", "age": 25, "is_student": False, "grades": [85, 90, 88]}json_string = json.dumps(python_dict, indent=2)print(json_string)# Writing JSON data to a filewith open('output.json', 'w') as file: json.dump(python_dict, file, indent=2)
The indent
parameter is optional but useful for creating human-readable JSON output. It specifies the number of spaces to use for indentation. When working with more complex data structures or custom objects, you might need to use the default
parameter of json.dumps()
to specify how to serialize objects that aren't natively supported by JSON.
Advanced JSON Manipulation: Tips and Tricks
As you become more comfortable with basic JSON operations in Python, you'll want to explore some advanced techniques to make your code more efficient and powerful. Here are a few tips and tricks to take your JSON manipulation skills to the next level:
Use the
default
parameter for custom serialization: When working with custom objects, you can define a function to specify how they should be serialized to JSON.Leverage
json.tool
for pretty-printing: Python'sjson.tool
module provides a command-line interface for formatting JSON data, which can be useful for debugging or presenting data.Explore alternative libraries: While the built-in
json
module is sufficient for most tasks, libraries likesimplejson
orujson
can offer performance improvements for large-scale applications.Handle encoding issues: When working with JSON data that contains non-ASCII characters, be sure to specify the correct encoding to avoid errors.
Use JSON schema validation: For applications that require strict data validation, consider using JSON schema libraries to ensure your data conforms to a predefined structure.
Practical Applications: JSON in the Real World
Understanding JSON manipulation in Python opens up a world of possibilities for data-driven applications. At Evomi, we've seen firsthand how crucial these skills are for businesses looking to leverage data intelligence and web scraping. Our clients use Python and JSON manipulation for a variety of purposes, including:
Extracting structured data from web APIs for market research
Parsing and analyzing large datasets for business intelligence
Building custom data pipelines for SEO optimization
Creating robust configuration systems for scalable applications
By mastering JSON manipulation in Python, you're not just learning a technical skill – you're equipping yourself with the ability to work with the lifeblood of modern data-driven businesses. Whether you're scraping web data, integrating with third-party APIs, or building your own data services, these skills will prove invaluable.
Conclusion: Empowering Your Data Handling Capabilities
JSON manipulation in Python is a fundamental skill for any developer working with data in today's interconnected digital landscape. From parsing API responses to creating data-rich applications, the ability to effortlessly work with JSON will make you a more effective and efficient programmer.
As you continue to explore the world of JSON and Python, remember that practice is key. Try working with different types of JSON data, experiment with more complex structures, and don't be afraid to dive into real-world applications. The more you work with JSON, the more natural and intuitive it will become.
At Evomi, we're committed to helping businesses leverage data intelligence and web scraping technologies to gain a competitive edge. Our range of proxy solutions, including Residential, Mobile, and Datacenter options, are designed to support your data collection and analysis needs. Whether you're just starting out or looking to scale your data operations, we offer flexible plans and a free trial to help you get started.
Remember, the world of data is vast and ever-changing. By mastering JSON manipulation in Python, you're taking a significant step towards becoming a data-savvy developer ready to tackle the challenges of modern software development. Keep learning, keep experimenting, and most importantly, keep building amazing things with your newfound skills!
